Metadata API
The Revenew Metadata API enables you to pass additional context about your payments to Revenew without needing to integrate directly. This low-code solution avoids costly development effort, streamlines your integration and provides a mechanism to further enrich your experience within Revenew products.
How it works
The Metadata API leverages a feature commonly provided by PSP Payment APIs as a conduit for additional information about your payments. Metadata fields are key-value pairs such as some_key=some_value
.
When Revenew receives payment notifications from your PSP, we decode any Revenew-specific metadata fields and turn them into instructions or structured data within the Revenew product.
Specifying Recipient instructions
If you use Revenew Boost to manage your seller (or more broadly, recipient) payments, or if you orchestrate them outside of the standard PSP payment flow, we need some basic information about each recipient in order to reflect your margins correctly within Revenew Clarity and optionally to orchestrate recipient payments via Revenew Boost.
Metadata API Recipient Fields
The table below defines the fields that can be used to describe the recipients of funds from a single payment.
Field | Description | Required | Type | Default | Example |
---|---|---|---|---|---|
id | Your PSP's identifier for the recipient | Yes | string | acct_1MjKupR478UofHYg | |
name | The name of your recipient, displayed in the Revenew Web App | No | nullable string | Brew Clothing | |
amount | The gross amount allocated to the recipient, in the minor unit (cents) of the presentment currency | No | nullable integer | Defaults to the full presentment amount | 10000 |
fee_amount | A fixed fee to charge the recipient, in the minor currency unit (cents) | Yes, unless fee_percent is specified, or using Boost | nullable integer | 150 | |
fee_percent | A variable percentage fee (between 0.00-100.00) to charge the recipient based on the presentment amount after conversion to your balance currency | Yes, unless fee_amount is specified, or using Boost | nullable decimal | 4.5 | |
currency_source | Whether to charge fixed fees in the presentment (presentment ) or balance (balance ) currency | No | nullable string | presentment | presentment |
fee_desc | A reference for the fee, displayed in the Revenew Web App | No | nullable string | Your Platform Fee | |
provider | The provider where the recipient account is located. Defaults to the PSP being used to process the payment. Possible values: stripe , airwallex , custom | No | nullable string | airwallex | |
billing | Indicates whether these fees are charged immediately (immediate ) or are deferred (deferred ) and paid later via an invoice. | No | nullable string | immediate | deferred |
Each recipient instruction is passed as a single metadata field in the following format with the details encoded or serialized according to each supported PSPs requirements:
rev_recipient_{index} = [serialized instructions]
The index
parameter is only used to ensure each metadata field is unique within the metadata object, which is typically a requirement of the PSP's API, for example:
{
"metadata": {
"rev_recipient_1": "...",
"rev_recipient_2": "..."
}
}
Examples
Variable Fee
In this example we have a 100.00 USD
payment allocated to a single recipient who is charged a fee of 10%
with a deferred billing cycle:
id: acct_1MjKupR478UofHYg
name: Brew Clothing
amount: 10000
fee_percent: 10
billing: deferred
Blended Fee
In this example we have a 100.00 USD
payment allocated to a single recipient who is charged a variable fee of 5%
as well as a fixed fee of 1.00 USD
:
id: acct_1MjKupR478UofHYg
amount: 10000
fee_amount: 100
fee_percent: 5
Blended Fee, multi-currency
In this example we have a 120.00 EUR
payment allocated to a single recipient who is charged a variable fee of 4.5%
as well as a fixed fee of 1.00 USD
:
id: acct_1MjKupR478UofHYg
amount: 12000
fee_amount: 100
fee_percent: 4.5
Note: Variable fees are always calculated based on the exchange rate used for the conversion by your PSP.
Using Stripe Connect
When using Stripe, serialize the Recipient Instruction as JSON
Stripe Connect payments are typically initiated by creating a Payment Intent. The Payment Intents API allows you to pass arbitrary information via the metadata
field.
If using Boost to handle the calculation of recipient fees and transfers, or if you use the Separate Charges and Transfers flow, add each recipient to the metadata object when creating the Payment Intent.
Using cURL
curl --request POST \
--url https://api.stripe.com/v1/payment_intents \
--header 'Authorization: Basic [Stripe API Key]' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data amount=10000 \
--data currency=usd \
--data 'metadata[rev_recipient_1]={ "id": "acct_1MjJWnQx0avvfgud", "name": "Brew Clothing", "fee_percent": 5, "fee_amount": 100 }'
Using NodeJS
Before:
const stripe = require("stripe")("[Stripe API Key]");
const paymentIntent = await stripe.paymentIntents.create({
amount: 10000,
currency: "usd",
automatic_payment_methods: {
enabled: true,
},
});
After:
const stripe = require("stripe")("[Stripe API Key]");
const paymentIntent = await stripe.paymentIntents.create({
amount: 10000,
currency: "usd",
automatic_payment_methods: {
enabled: true,
},
metadata: {
rev_recipient_1: JSON.stringify({
id: "acct_1MjJWnQx0avvfgud",
fee_percent: 5,
fee_amount: 100,
}),
},
});
How Revenew uses recipient instructions
How we use recipient instructions depends on the products and features you have enabled.
When not using Boost (Default)
If you don't use Boost but instead execute your recipient transfers outside of your PSP's standard payment flow, Revenew will match these transfers and any recipient instructions provided to us in order to calculate your margins correctly.
This is particularly important if you split your recipient payments into multiple transfers so that we can determine whether each recipient has been fully or partially paid.
When using Boost
If you use Boost, once a payment has been successfully processed we will automatically calculate your recipient fees according to the pricing profiles you have configured within Revenew and orchestrate the necessary transfers of funds via your PSP.
Using different payout providers
If you use a different provider to manage payouts to your recipients you must specify the provider
field for each recipient. Revenew will automatically attempt to verify and retrieve information about the recipient to provider a better experience within the Revenew Web App:
{
"id": "acct_KpWxgKnTNWW8xnysXOBUlQ",
"name": "Brew Clothing",
"fee_percent": 5,
"fee_amount": 100,
"provider": "airwallex"
}
If you are using your own internal systems to manage payouts (such as aggregating multiple providers internally), use the value custom
so that Revenew will skip the verification check.
You can also specify the name
of the recipient for display purposes. For example:
{
"id": "custom_1234",
"name": "Brew Clothing",
"fee_percent": 5,
"fee_amount": 100,
"provider": "custom"
}
Specifying Payer & Revenue Categories
Revenew supports attributing payments for subscriptions and invoiced fees to your existing customers, providing you with a complete view of how your customers are performing.
Handling Subscriptions
Revenew automatically supports Stripe Billing subscriptions, correctly categorizing these payments as Subscriptions within Revenew. To associate Stripe Billing payments with an existing customer, some metadata needs to be provided on the Stripe subscription.
In Revenew, a customer who is paying for a subscription or an invoice is called a payer. To add a payer to a subscription, a rev_payer
field needs to be added to the Stripe metadata of the subscription with the customer's Stripe account.
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud"
}
}
Adding Metadata via the Stripe Dashboard
Metadata can be added to subscriptions within the Stripe Dashboard without any coding required.
- Navigate to Subscriptions.
- Click on the subscription you want to update.
- Scroll down to the Metadata section and click on the edit icon.
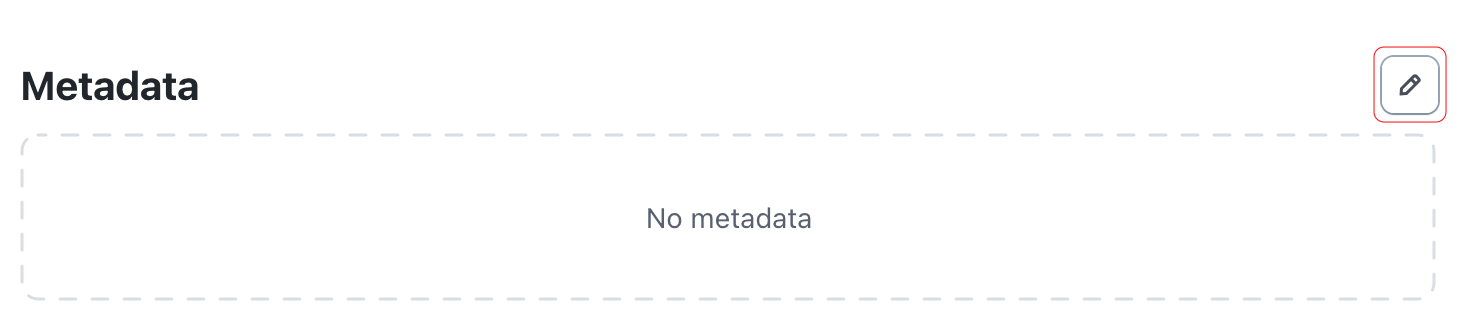
- Enter
rev_payer
as the Key and the Stripe Account related to this customer.
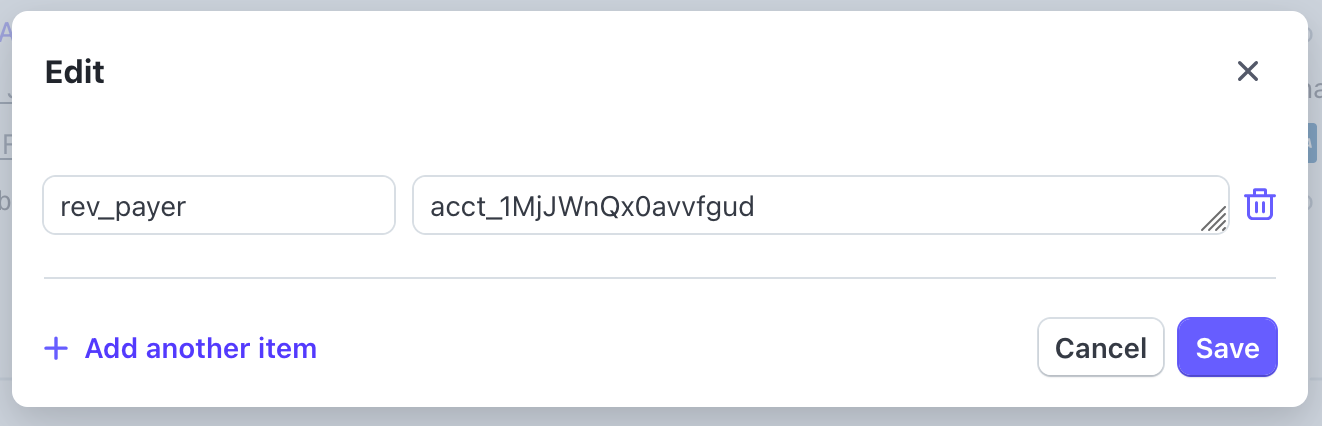
- Click Save
The next time this subscription is charged, it will be linked in Revenew with the customer associated with this Stripe Account Id.
Adding Metadata using Curl
Metadata can also be added to subscriptions using the Stripe API.
curl https://api.stripe.com/v1/subscriptions/sub_1MowQVLkdIwHu7ixeRlqHVzs \
--header 'Authorization: Basic [Stripe API Key]' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data 'metadata[rev_payer]=acct_1MjJWnQx0avvfgud'
Non-Stripe Billing Subscriptions
Third-party subscription solutions are also supported but require additional metadata added to the payment to classify it as a subscription.
Adding rev_category_subscription
to the metadata, in addition to the rev_payer
metadata, will indicate to Revenew that this is a subscription payment.
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud",
"rev_category_subscription": ""
}
}
If the client you are using does not support empty values, you can also specify the full payment amount in minor units (cents).
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud",
"rev_category_subscription": "10000"
}
}
If only part of the payment is related to a subscription, then the amount in the presentment currency for the subscription can be added to the metadata.
For example, a $100 payment, where $80 is part of a subscription and $20 is for a product, would be represented as:
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud",
"rev_category_subscription": "8000"
}
}
Note: The amount should be in minor units (cents).
Invoiced Fee Payments
If your customers are gross settled and later invoiced for incurred fees, this can be represented in Revenew using revenue categories.
Recipient payments will need to have metadata associated with them for us to calculate your margins correctly (see Specifying Recipient Instructions). If recipients are paid gross and then later invoiced for fees, you can set billing
to deferred
to indicate this.
id: acct_1MjKupR478UofHYg
name: Brew Clothing
amount: 10000
fee_percent: 10
billing: deferred
This will allow margins to be calculated correctly even if the recipient received 100% of the funds at the time of the payment.
Invoice payments for fees are handled differently in Revenew. Recipient payments with metadata have already had the fee included in your net revenue and margin calculations. Therefore, subsequent invoice payments for fees should not impact your GMV, net revenue, or margin calculations. The PSP fees associated with these invoice payments should contribute to the PSP fees of your embedded payments.
To categorize a payment as a fee payment, a rev_category_fee
metadata item should be added.
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud",
"rev_category_fee": ""
}
}
Using curl:
curl --request POST \
--url https://api.stripe.com/v1/payment_intents \
--header 'Authorization: Basic [Stripe API Key]' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data amount=10000 \
--data currency=usd \
--data 'metadata[rev_payer]=acct_1MjJWnQx0avvfgud' \
--data 'metadata[rev_category_fee]='
If the client you are using does not support empty values, you can also specify the full payment amount in minor units (cents).
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud",
"rev_category_fee": "10000"
}
}
This metadata can also be added directly to the invoice instead of the payment if you are using Stripe Billing.
curl --request POST \
--url https://api.stripe.com/v1/invoices \
--header 'Authorization: Basic [Stripe API Key]' \
--header 'Content-Type: application/x-www-form-urlencoded' \
--data customer=cus_RdjPO5edgC10Bl \
--data auto_advance=false \
--data 'metadata[rev_payer]=acct_1MjJWnQx0avvfgud' \
--data 'metadata[rev_category_fee]=10000'
If only part of the payment is an invoice for fees, then the amount can be specified in the metadata. The amount should be indicated in the minor unit (cents) in the presentment currency.
For example, a $100 payment, where $80 is an invoice for fees and $20 is for a subscription, can be represented as:
{
"metadata": {
"rev_payer": "acct_1MjJWnQx0avvfgud",
"rev_category_fee": "8000",
"rev_category_subscription": "2000"
}
}